Object Oriented Programming in Python Series
PART II
Hi there!, welcome to the part two of my object oriented programming in python series. in the previous article, I talked about classes and how to create and instantiate them in python. Also, I differentiated between public and private attributes and also discussed instance and class attributes.
In this article, we will talk about methods and how to create them. we will also talk about the different methods we have and how to create them using “decorators”, which are python functions for wrapping other functions and methods. So, let’s dive in, shall we?
Methods in python are also functions, but are bound to the scope of classes. What I’m saying, is that methods are simply functions that resides in classes. These functions are otherwise known as the “behaviors” of classes that define them. They are the actions that those classes perform. enough talk, let’s dive into how to create them and use them.
We will build a class car and give it attributes name, price and gear. This will be a simple class as I want us to only get how methods work, later on in this series, we will build more complex classes and even talk about enums(enumerations).
Instance Methods
These methods are functions that are unique to each instance. i.e, these methods on creation of concrete objects are bound to those objects and do not interfere with other methods of other objects.

From the above image. you will notice that each student has its own getAverage() method which calculates the average for each student. getAverage() in this instance is an instance method which is bound to each concrete student object. Let’s see below example of instance method for our Car class
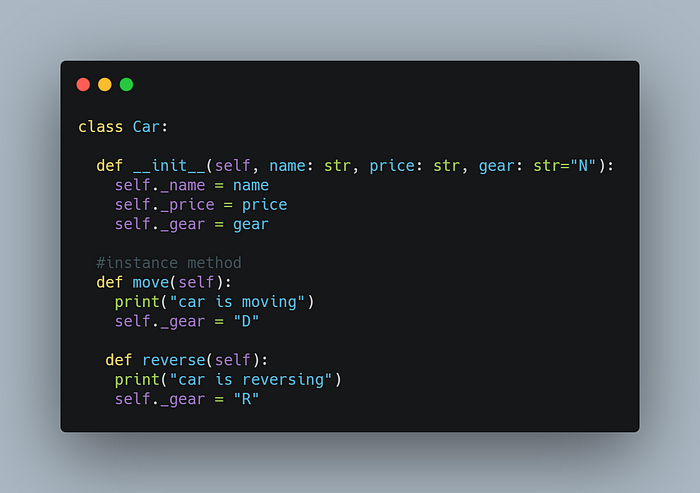
Our Car class defines two methods, move() and reverse(). In the definitions, notice there is an argument “self”, this self argument refers to an instance of the Car class, thus allowing the methods to modify the gear attribute. When these methods are invoked or called, the lines of code inside the body of those functions are executed and the gear attributes is changed according to the method called. Let’s instantiate our class and perform few actions

Our Car object performs just two actions, move and reverse. You can modify it on your end to park and do other things.
There’s one thing to note here. When we defined the methods, we gave them a parameter self, but when calling, we did not pass any parameter, why? because self refers to the car object we created which is an instance of the Car class we defined.
Class Methods
unlike the methods we discussed earlier, class methods are also actions performed by classes, BUT, these actions are bound to the class itself. This means these methods are linked to the class definition and when the class is instantiated, these methods are shared by all instances of that class.
To define these methods, the “@classmethod” decorator is called on the method we which to define as a class method. One difference is that it takes in cls as parameter instead of self. cls refers to the class as we can’t use the class keyword again. This methods also allows the modification of instance and class variables.
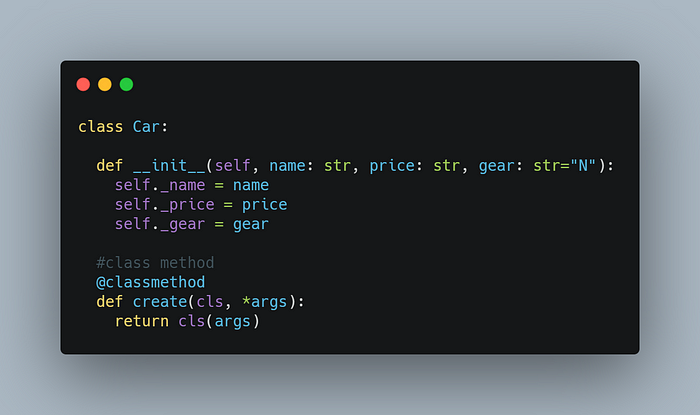
What happens when we call this method? This method can be called directly on the Car class or on an instance object, and it will create and return a new Car object which can be instantiated.
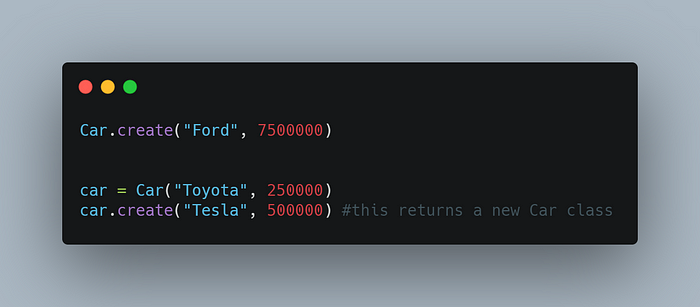
class methods are useful when we are building parsers as they allow us create new parsers with custom definitions.
Static Methods
This is the last one among the different methods available in python. This method works like an ordinary pure function. it does not access the instance variables of the class it is defined in and can only use variables passed as arguments.
These methods do not interfere with the class and they form something like a symbiotic relationship where the class provides shelter for them and in turn they serve as utility functions for the class.
They are created using the “@statismethod” decorator on the preferred method and do not take in either self or cls as arguments, rather normal arguments.

calling the check_gear method with an argument “gear” will return a boolean telling whether the passed gear is part of valid gears for the car.
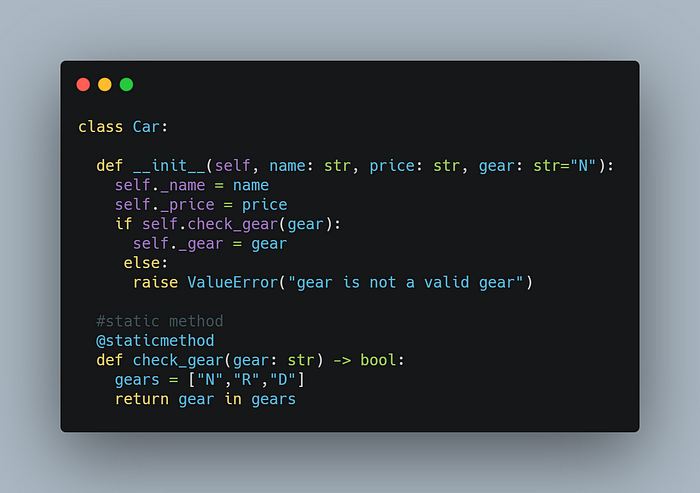
the constructor will raise an error if the gear passed is not valid and it will create an instance variable gear otherwise.
CONCLUSION
We have talked about the various methods available for use when building our classes, and also how each method works.
Instance methods are bound to instances while class methods are bound to classes and the last one is merely a resident inside the class scope
Below gives a comparison between each method
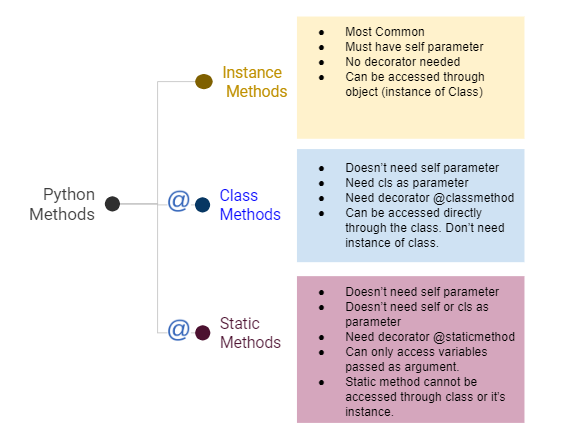
You can play around with these methods to further solidify your knowledge.
Thank you for reading, and in the next part, we will talk about properties and how they work. Thank you! and leave a comment